Ruby on Rails 7
“I'd nearly given up trying to learn Rails. Every course or tutorial out there left me confused and frustrated.”
Every time we hear this (and we hear it almost daily!) it breaks our heart.
It's sad because the Ruby on Rails framework was intentionally designed for optimal programmer happiness and beautiful code! And yet, when it comes to learning Rails, you're left spinning your wheels.
Wrong!
Learning Rails should be filled with many wonderful "Aha!" moments. But there's a good chance other courses spoiled that by either (unintentionally, of course) treating you like a 6-headed superhuman or a half-brained dimwit.
If you really had 6 brains, you could juggle multiple concepts at the same time. And yet, in our 21 years of teaching, we've only run across such creatures in sci-fi movies. In reality, we all just have one brain. It can multi-task when it's in survival mode. But in learning mode, your brain benefits from deliberate focus.
A Rails course that shoves in non-Rails topics and claims to give you "more value for your money" is really saying "we're going to throw a bunch of stuff at you and hope like crazy some of it sticks."
You know what really ends up sticking? The idea that Rails is magical and mysterious. And now you're really stuck because you can't start or maintain a Rails app with any degree of confidence.
So other Rails courses take the opposite approach. They assume you're a dimwit who's content with shallow explanations, quizzes that give you a false sense of understanding, and a pocketful of points. Satisfying? Hardly!
All those points don't matter if getting your app from idea to deployment leaves you in despair over time and opportunity lost.
Your dreams are dashed.
And who could blame you?!
“I was almost giving up on Rails. But now I see that the Rails "magic" is totally understandable! This was a major relief and accomplishment, because no other Rails course or book (online or offline) got me going.”
André Garcia
“Thank you very much for this fantastic course! I learned more from your course than the 6 months I spent at a Rails coding bootcamp. I loved the visual representations and the very very clear explanations.”
Aya-Julie Koffi
How much more rewarding (and fun!) would it be to pop open your code editor next week and...
- Build your own app from scratch, and be proud of the result
- Confidently jump into an existing Rails app
- Enjoy a smooth workflow for building apps faster, and better
- Laugh in the face of error messages (you know how to fix them!)
- Code, think, and truly "get" the Rails way
“I completed a few Rails tutorials before but this course contained more lightbulb moments than the rest of them put together. The explanations were clean, clear, and precise, and breaking things down into diagrams really helped. After completing your course I can now build pretty much whatever I want in Rails.”
Jonathan Mundy
The same can be true for you!
Once you understand how all the pieces fit together, you can build whatever you want with Rails.
For 16 years we've been helping developers just like you move from confusion to confidence with Rails. One concept at a time. One clear explanation at a time. One "AHA!" moment at a time.
In our Rails course, we build a full-featured app from start to finish, step-by-step. For each new topic you:
- Watch a short live-coding video
- Visualize the concepts with rich animations
- Practice in a project-based exercise
- "Aha, now I get it!"
- Repeat for next concept...
You see every move, every change, every refactoring first hand. It's as if we're sitting down together building a Rails app. And we do our best to make it fun and friendly!
The live-coding videos (8 hours total) are laser-focused on advancing the app one feature at a time. Each video is:
- easy to digest (54 bite-sized videos in total)
- straight to the point without any rambling or fumbling
- perfectly paced so you're not left behind or nodding off
- streamable with English subtitles and also downloadable
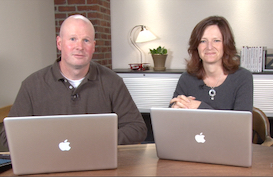
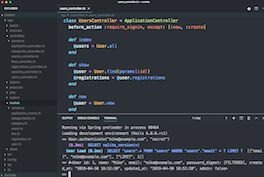
“I think we've all wasted time on silly tutorials and websites that promised quick and easy results but left us more confused than when we started. In this course I never once got lost in the Rails magic.”
Hunter Stewart
Seeing code is hardly enough. You also need to see the entire system at work. Each of the 45 visual explanations throughout the course give you:
- a solid mental model for how everything fits together
- a visual explanation that takes the mystery and magic right out of Rails
- the key to becoming the magician instead of the mystified!
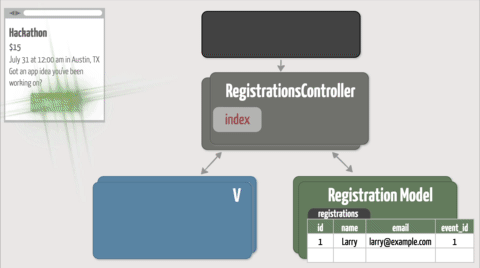
The visual explanations with animated diagrams were an absolute game-changer for me. As a visual learner, I never really grasped the MVC concept before this course. They really helped me understand what was going on. Now, I understand the whole flow, from URL to HTML. I almost became convinced I couldn't learn Rails. Thankfully I came upon your course!
Nathan Roise
Here's the kicker: you need deliberate and directed practice to make all this permanent. That's why you also get a 52-chapter workbook. Each video has a companion workbook chapter with exercises where you'll:
- work through step-by-step tasks with inlined hints and solutions
- build a separate, different application than in the videos
- reinforce and cement what you learned in a different context
You not only get hands-on experience, but also mind-on experience. And that's what makes it all "stick". On average, developers take a total of 26 hours to read the workbook and complete all the exercises.
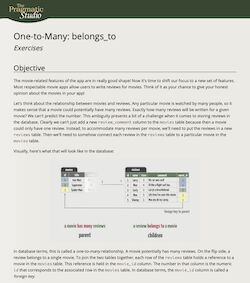
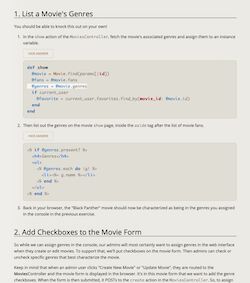
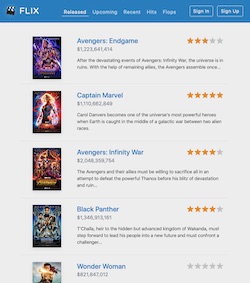
I like how the exercises are separated from the videos. It's really helpful to watch you build one app in the video and then build a different app on my own afterward. One thing that bothers me about other video tutorials is that I have to constantly start and stop them in order to code along with the video. But with yours, I can actually focus on what you are doing and then implement the concepts in the exercise after the video. I was able to build a ton of features into an existing Rails app at work after taking this course.
Andrew Talle
You also get:
- 40+ PDF cheat sheets summarizing syntax, concepts, conventions, and shortcuts
- Starter files and versions of the code for each video and exercise
- The final source code for 2 full-featured Rails apps
I felt like I had a ton of 'A-HA!' moments... What really made things stick for me in this course was the fact that I wasn't building the same exact thing that the instructors were... The animated graphics show you how different parts are moving around behind the scenes and effecting each other. The visuals definitely helped me to understand things more in depth.
Matt Steele
Get started today!
No monthly subscription or time limit. You own it for life.
Questions? Email Mike and Nicole.
There's no risk. This course is 100% guaranteed for an entire 30 days. If you find it's not a good fit for you, we'll be happy to refund your money.
Live in a country where our courses aren't affordable? We offer purchasing power parity discounts.
“Of all the courses I looked at out there, your course is the highest quality (and the best price) I found—hands down! At first look, I thought 'Whoof, that's a chunk of change to pay for a course.' After the first week, I thought this is definitely worth the cost. And now I am thinking this is absolutely the best bargain. I cannot summon enough superlatives to describe how much I have learned and how much more confidence I have in my Rails skills. I have learned more with your course than any other single resource I've tried.”
Ryan Benedetti
“I've taken quite a few Rails courses and I've read quite a few books on Ruby/Rails. Many of them confuse you with extra details and digress too often to be comprehensible. In contrast, in this course the entire process of building an app is presented in a step-wise and fulfilling way. Bottomline: Stop going through random books and videos. Mike and Nicole's courses will save you time and frustration in the long run and they are well worth 10 times the price.”
Jay Luong
Meet Us In This Video
Course Outline
Videos just the way you like 'em: easy to digest, straight to the point, and paced just right.
Welcome! After taking a peek at the apps we're going to build in this course, we'll help you get your development environment set up so you can start building Rails apps.
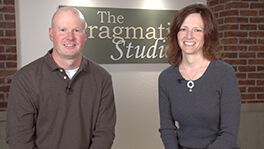
To make sure everything is up and running smoothly, we start by generating a skeleton Rails app and then get a quick lay of the land.
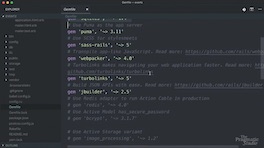
Rails has some strong opinions about how web apps should be designed. You've probably heard about the MVC design in the abstract, but we break it down in practice so you understand where to put your code and the benefits of a decoupled design.
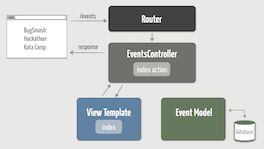
Well-designed models are the foundation of any good Rails app. Active Record is the object-relational mapping library that Rails uses to connect your models to your database tables. We don’t yet have a web interface for our app, so instead we see how to create, read, update, and delete records in the database via the console.
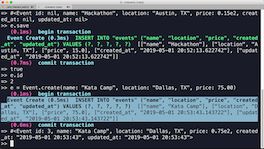
Now that we have some events stored in the database, the next step is to display them on the index page. To do that, the model, view, and controller must all work seamlessly together.
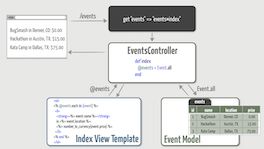
The details about our events are looking a little sparse. So we add more attributes, which requires knowing how to manage the database schema with migrations.
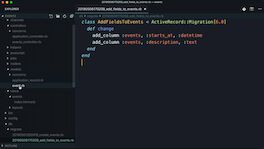
The new event attributes are now displayed on the index page, but their formatting leaves a lot to be desired. We use both built-in and custom view helpers to give our app a lift and make things more reusable. We also answer the question: "Where does business logic belong?"
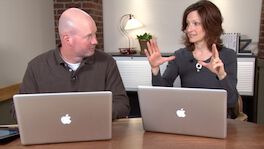
To give our app a consistent look and feel, all the common layout elements need to live in one definitive place. Because when it comes to the layout of your app, consistency never goes out of style.
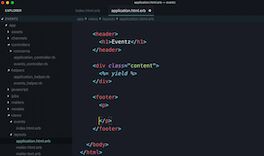
It's always more enjoyable to work on an application that has some style and images. Adding in these assets gives us an opportunity to learn about the Rails asset pipeline.
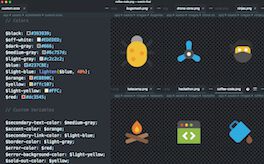
The Rails router receives incoming requests and, depending on a set of rules, dispatches these requests to an appropriate controller action. We start by defining a route that recognizes requests to show an event's details.
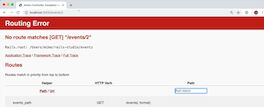
In addition to being able to map requests based on defined routes, the router can also generate URLs that match these routes. We learn how to use route helper methods to generate links to navigate between pages.
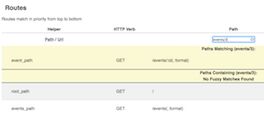
Rails has a bunch of conventions to help you create robust and friendly forms with minimal code. We unveil the "magic" as we design a form for editing records, store submitted form data in the database, and display updated records.
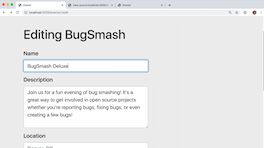
Reinforcing what we learned about forms for editing records, we tackle creating records as we continue to implement the full range of CRUD actions using resource routes.
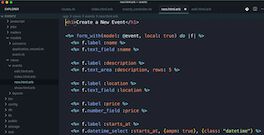
Crafting good Rails apps isn't just about implementing features that work as advertised. Good Rails apps also have clean, well-organized code. To that end, we learn how to use partials to structure the view layer into reusable, manageable chunks.
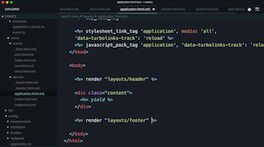
We finish up implementing the resource routes by deleting records. Here's the cool part: All resources follow the same routes and conventions. So at this point in the course, you can confidently build a CRUD interface for any resource!
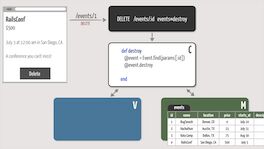
Next up, we need to fetch a subset of events from the database and order them in a meaningful way. Thankfully, Active Record has a rich query interface that insulates us from having to write raw SQL to query our data. Master these query methods and you can slice and dice your data with ease, regardless of which database you use.
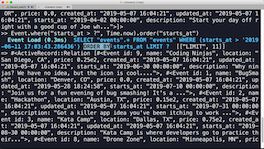
To meet a new requirement, we need to add new fields to a database table. Time for a new migration! And anytime you migrate the database, you also need to think through the ripple effects. Come along as we work through all the steps to accommodate a new migration.
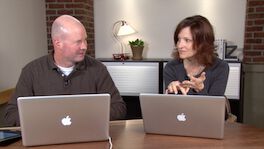
To prevent bad (invalid) data from making its way into the database, we add a variety of model validations and explore how they work in detail.
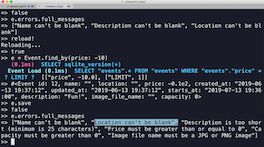
With our model validations in place, we're ready to handle and display any validation errors when submitting form data. You'll come away with a solid strategy for ensuring the integrity of your application's data while providing actionable feedback in the user interface.
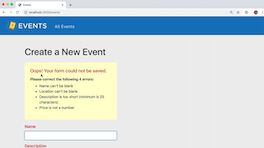
While on the topic of user feedback, we have a few cases where we need to flash a stylish message up on the page. It's common for web apps to flash messages between requests, and so Rails makes it easy.
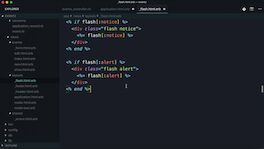
Up to this point, we've been focused on one resource: events. Now we want to let folks register for events. To do that, we first create a registration resource and then start designing a one-to-many relationship between events and registrations. One of the most powerful features of Rails is the ability to easily create these types of associations. But knowing the magic incantations isn't enough. To be empowered, you also need to how what goes on behind the scenes.
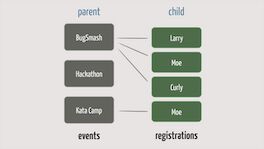
Now it's time to flip over to the other side of the one-to-many association. An event has many registrations, otherwise it wouldn't be a very exciting event. This video shows you how all the dots are connected!
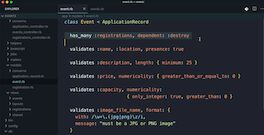
Registrations only make sense in the context of their associated event. To mimic that one-to-many relationship in our routes, we nest registration resources within an event resource. This is a common design technique that you'll definitely want to have in your arsenal!
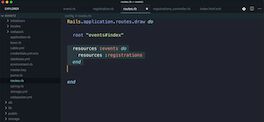
How do you design a form for a one-to-many relationship so you can create child records that are associated with their parent? Answering this gives us an opportunity to apply (and reinforce) everything we've learned so far.
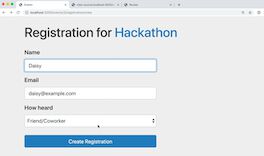
Once you start making associations between models and collecting data, you'll likely discover some interesting things you can do with it. For example, what does it mean for an event to be sold out? What's the average star rating for a movie? What determines if it's a cult classic? The code that answers those type of questions is part of the business logic of your domain. And knowing where to put that code is vital to designing a maintainable application.
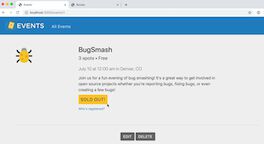
User accounts are a central part of any web application. By learning how to design a basic account management and authentication system from scratch, you'll know exactly how it works. With that understanding, you'll have the confidence to roll your own custom solution or integrate (and troubleshoot) a third-party solution. We start by designing a solid User model that securely stores passwords using best-practice Rails conventions.
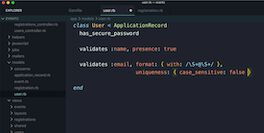
Now we're ready to design a signup form so users can create accounts and a profile page that displays their account information. To make the sign-up process more friendly, we also add a custom route.
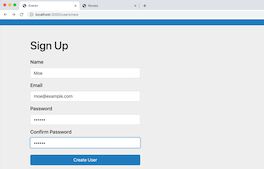
Obviously, users also want to be able to edit their account information, and (dare we say) perhaps even delete their account. Then, with a complete UI for user accounts in place, the stage is set for authentication and other account-related features.
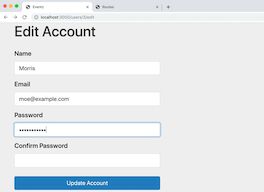
Now that users can create an account, the next logical step is to let them sign in. We start by designing a sign-in form.
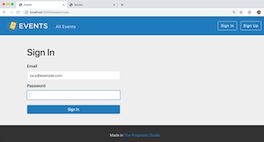
Next, we confirm you are who you say you are by authenticating a user given their sign-in credentials.
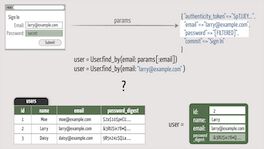
Once authenticated, we use a session to identify the current user as they navigate from page to page.
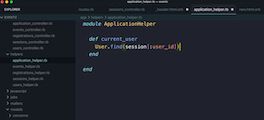
And of course no authentication system would be complete without a way to sign out!
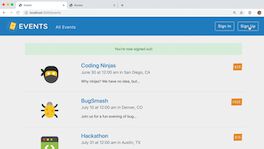
Now that we know whether a user is signed in or not, it's time to start restricting access to parts of the application. This process is commonly referred to as authorization. And while authorization rules vary widely depending on the nature of the application, once you understand the basic technique you can apply it as you see fit.
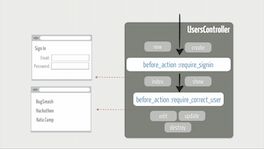
Only super-special users we trust should be able to perform highly-sensitive actions in our app. So we need a way to distinguish admin users from regular users, and restrict access accordingly.
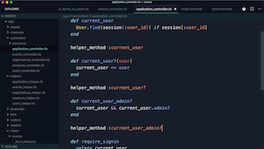
With user accounts and authorization in place, we can now streamline processes within the app for currently signed in users. To do that we create our first many-to-many association which connects a user to an event using a join model and a form.
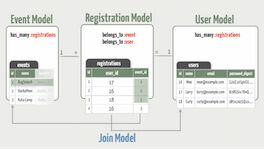
Rails has powerful conventions to help you manage many-to-many associations, but the conventions alone only take you so far. It's up to you to model the associations with the full stack in mind: from the database tables all the way through to the user interface. We look more in-depth at these details in a second many-to-many association.
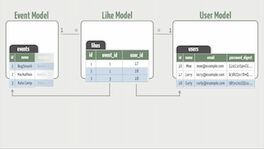
In the absence of a direct relationship between two models, which is the case with many-to-many relationships, how do you efficiently traverse between them? Thankfully, Rails offers a really convenient way: through associations. They can seem a bit magically at first, but we quickly dispel any confusion.
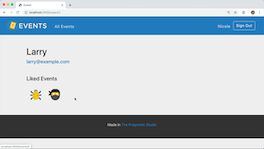
Many-to-many associations are really common, so to continue building your confidence we design a third many-to-many. This time we use a through association in the models and a collection of checkboxes in the user interface to assign multiple categories to events and multiple genres to movies.
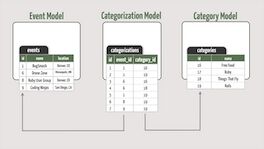
One approach to defining a custom query is to write a class-level method. But a more idiomatic and concise way to write a custom query is in a declarative style using the scope method. Doing so lets us slice and dice our events and movies into all sorts of interesting categories. With our scopes in place for specific criteria, it's time to show them off. We set up custom routes so that users can easily filter what they're looking for.
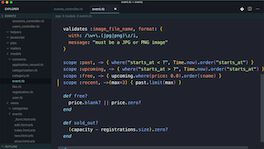
URLs are the user interface of the web. We browse to them, bookmark them, post them to Twitter, email them to friends, and share them in other ways. And don't forget the search engines that crawl them! As part of your app's user interface, URLs require intentional design. One common technique is to include meaningful and friendly names (rather than numeric ids) in resource URLs. We show you exactly how to do that, exploring Active Record callbacks along the way.
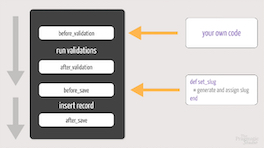
Putting your Rails app into production shouldn't cause fear and trembling. Deploying Rails apps has gotten a lot easier over the years thanks in large part to cloud services such as Heroku. We'll show you how to deploy your Rails app for the first time, and incrementally roll out application updates with ease!
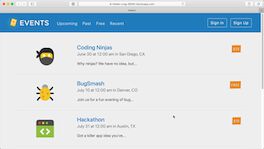
Rails has a built-in solution for letting users upload files. Active Storage facilitates uploading files to a variety of destinations, including cloud storage services such as Amazon S3, Google Cloud Storage, or Microsoft Azure. To get things started, we set up Active Storage to store uploaded images on the local disk in development. We also go behind the scenes to see how Active Storage manages uploads using two database tables and a polymorphic association.
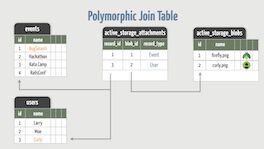
All the cloud-based storage services require a set of super-secret credentials to verify who we are. Here's the rub: we need a way to encrypt those credentials so we can safely deploy them along with the application code. And when the application runs in production, we need a way to decrypt the encrypted credentials so Active Storage can use them to communicate with our storage service. Thankfully, Rails has a tried-and-true way to do that.
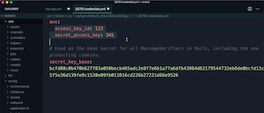
Finally we're ready to use Active Storage to store uploaded images on Amazon S3 in production! And from there, it's pretty straightforward to store uploaded files in any supported storage service. 🥳
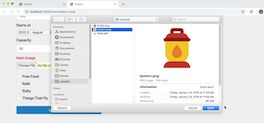
For Developers New to Rails
Who are already familiar with Ruby
To keep this course focused and paced just right, we assume you're comfortable with Ruby— it's the programming language you use to write Rails apps.
New to Ruby? No worries. Our comprehensive, beginner-friendly Ruby course will totally get you ready for Rails. We think it's the best course on Ruby that you'll find, and lots of folks agree!
Created with 💛 by
Mike and Nicole Clark
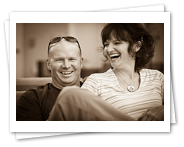
Howdy! We're the friendly couple behind The Pragmatic Studio.
We've been making courses for developers since 2012. It's a privilege to get to teach together and serve everyone who supports us.
We've been using Rails since version 1.0. In fact, Mike helped build and launch one of the first production Rails apps way back in 2005. Since then together we've built countless Rails apps, including the suite of apps that power this site and our custom video training platform. We still write Rails code almost every day, and we love it!
Mike co-taught the first official Rails course in 2006 which lead to us starting this little training company we call The Pragmatic Studio. He's also the author of Advanced Rails Recipes, co-author of Agile Web Development with Rails, and author of Pragmatic Project Automation.
This is the course we wish we had when learning Rails. One that's easy to jump into, doesn't get bogged down in syntax minutiae, and focuses on building a real Rails app. Nothing is held back: we tackle complex features by breaking down the concepts so everything makes sense. We hope you enjoy it!
💥 This Course Is A Hit! 💥
“This is the best Rails course hands-down! I've worked through several Rails courses and they don't even come close to explaining things as well as you do. You have the perfect balance of teaching the right amount of information such that I understood each new piece without any confusion. I've always felt like Rails has too much of a learning curve, but you've communicated its elegance and flexibility. If you put out more courses I will certainly come back each time to learn more from you. Again, out of all the resources I've worked through, nobody has come close to connecting with me in the way that you guys have. Bravo!” Thomas Neal
“Simply amazing. The best online course I have ever experienced. Nicole and Mike, you are the best!” Tony Barone
“It is the absolute best for a first dive into Rails! It teaches you the correct way to start a new Rails app...If you go through the whole course, [the] magic will be explained and you’ll know what the Rails framework does instead of passively look at it do things for you.” Nico Schuele
“I've tried almost every Rails learning site out there. While all of those resources taught me how to accomplish certain tasks in Rails, I felt there was a large gap between knowing how to do something vs. understanding what's happening at a deeper level. Your Rails course bridged the gap for me.”Michael Zsigmond
“I really love the quality of your videos and lectures, and the way it builds up an app. It's the best that I've taken!” Vita Dewi van Beurden
“This is the BEST Rails introduction anywhere on the web! I learned a ton in this course that I didn't learn from other sources online. I was immediately able to start putting together an application that has been stuck in my head for a long time.” Wassim Metallaoui
“This course along with your Ruby Programming course offers the best introduction to learning Rails for a beginning developer. I cannot think of any book or video series that does as good a job.” Eric Ricketts
“The main difference I have found after taking a lot of other Rails courses is that you explain things in greater detail which has helped me understand the 'whys'.” David Theroff
“It seems like there are a lot of courses and tutorials out there that are great if you already know how to program in another language, but there are none that I know of (other than Pragmatic Studio!) that is great for a complete beginner. After taking your Ruby course I feel like I have a solid foundation to stand on. Now, learning Rails has been a lot of fun instead of a lot of frustration.” Andrew Markle
“Thanks to everything I learned with you, I am about to land a new job in which I will be programming in Ruby on Rails. Thank you very much for that!” Miriam Tocino
“It's the perfect gateway to Rails. The major problem with learning Rails is the "magic". When you are a beginner it's hard to understand the mechanics behind it. This course has you do things by hand from the very beginning, which allowed me to finally understand the deep principles. Thank you!” Johan Watelet
“This is the best beginning Rails course I've found. I finally have a strong Rails foundation. Coming into the course, I had so many loose ends regarding how Rails worked, but I finished the course with the confidence to create my own app.” Brad Ballard
“The Rails course is leagues above some others I've tried. First of all, Mike and Nicole have skills as instructors, not just developers, so I didn't feel they were talking above my head. Also it's clear that the lessons and supporting files have been tested and edited -- because they work! The exercises in the example apps are very relevant and I left the course with a whole new level of expertise. I can't recommend this course enough!” Anne Richardson
“This Rails course is fabulous! I took a few other courses, but still felt really confused. I learned a lot, feel more comfortable with Rails, and really understand how controllers, models, and routes depend on each other. The explanations along with the illustrations were really fantastic. This is my favorite Rails course.” Dana Nourie
“The Ruby on Rails course from @pragmaticstudio was without a doubt one of the best online courses I've ever taken. So clear, fun and—dare I say—pragmatic!” Onur Özer
“This was an amazing course! The way each new concept is introduced on its own and the apps are built out gradually, never made it overwhelming to try applying the new concepts myself. The pacing was really well done and everything was thoroughly explained. The whole process really helped me retain everything.” James Groves
“I've taken other Rails tutorials but yours really filled in the gaps in my understandings. I can now truly say that I've taken a big step towards developing with Rails.” John Weir
“Our new hires are loving your online Ruby and Rails courses. In the past, our senior engineers taught new team members Rails on their own. But now with your courses, we are getting new engineers up to speed much easier and faster.” Eirik Holm, AppFolio
“I had tried other courses and had tons of issues with them. This is the first one that actually went quite smoothly and I felt like I was learning at a much faster rate. Highly recommended course!” David Hood
“Everything is great: the material, the teachers, the simple, concise, yet clear explanations in all the courses. I think these are the best Ruby and Rails online courses for people that want to jump into Rails. All of the courses are worth every penny!” Alfian Losari
“This course is fantastic! I had read a couple of Rails tutorial books and sort of understood what the example code was doing, but was having trouble sorting out how to build my own app. This course really filled in some missing pieces.” Terrence Talbot
“I highly recommend Pragmatic Studio's Rails Programming courses! The exercises allow you to build something more complex than a blog and do so by applying what was learned in the lessons rather than merely following along! This format really drove things home for me.” Bart Falzarano
“After trying many online Ruby on Rails courses across the Internet, this is the most thorough and enjoyable introduction available. Rhys Yorke
“A partner and I just released and started getting sales on an RoR app I created. I was able to build the prototype in a weekend! You all are a big reason I was able to do it. You have done a wonderful job of taking a lot of the 'magic' out of Rails.” Justin Seiter