What Is Absinthe?
April 04, 2019
(This is Part 4 of a series: Part 1, Part 2, Part 3)
Simply put, Absinthe is an Elixir implementation of the GraphQL spec. At its core, you give Absinthe a GraphQL document and a schema (written in Elixir, of course) and you get you back a JSON result. Combine that with the superfast Phoenix framework and you’ve got a robust, high-performance GraphQL API!
This 1-minute animation shows how everything fits together:
In summary, here’s how you run a GraphQL document (a query in this case) using Absinthe:
document = """
query {
places {
id
name
location
}
}
"""
result = Absinthe.run(document, GetawaysWeb.Schema)
Here’s an example Absinthe schema:
defmodule GetawaysWeb.Schema do
use Absinthe.Schema
alias GetawaysWeb.Resolvers
query do
@desc "Get a list of places"
field :places, list_of(:place) do
resolve &Resolvers.Vacation.places/3
end
end
mutation do
@desc "Create a booking for a place"
field :create_booking, :booking do
arg :place_id, non_null(:id)
arg :start_date, non_null(:date)
arg :end_date, non_null(:date)
resolve &Resolvers.Vacation.create_booking/3
end
end
subscription do
@desc "Subscribe to booking changes for a place"
field :booking_change, :booking do
arg :place_id, non_null(:id)
config fn args, _info ->
{:ok, topic: args.place_id}
end
end
end
end
Internally, at the heart of Absinthe is a pipeline of transformations that parse, validate, and execute GraphQL documents against the schema:
document
|> parse
|> validate
|> execute
The pipeline is easily configurable so you can add your own steps. And as you’d expect from Elixir, Absinthe is robust and blazing fast!
But Absinthe goes beyond just an implementation of the GraphQL spec. While the core Absinthe package isn’t tied to any particular Elixir web server, it includes specialized packages for integrating Absinthe into a Phoenix web application.
There’s an absinthe_plug package that handles GraphQL documents sent over HTTP to a Phoenix endpoint. Here’s an example of how to configure the Phoenix router to forward GraphQL requests to the Absinthe.Plug
plug which runs any GraphQL documents against the specified schema:
forward "/api", Absinthe.Plug,
schema: GetawaysWeb.Schema
And there’s an absinthe_phoenix package that supports GraphQL subscriptions over Phoenix channels.
Plus Absinthe includes a bunch of advanced features for performance tuning your queries, whether your data source is Ecto talking to a local database, or an external data source.
Now imagine what would happen if you incorporated all that in a full-stack application? Well, that would be pretty sweet…
Unpack a Full-Stack GraphQL App Layer-By-Layer
Learn what it takes to put together a full-stack GraphQL app using Absinthe, Phoenix, and React in our Unpacked: Full-Stack GraphQL course. No need to piece together solutions yourself. Use this application as a springboard for creating your own GraphQL apps!
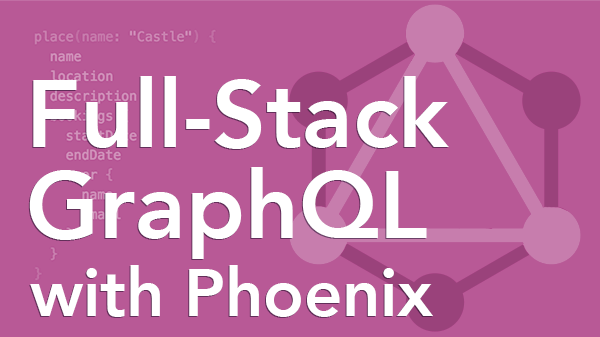