What Does A GraphQL API Know?
March 21, 2019
(This is Part 3 of a series: Part 1, Part 2, Part 4)
We know from the previous videos that a GraphQL API is a special kind of API that supports queries, mutations and subscriptions. But we can’t just throw any old query, mutation, or subscription at a GraphQL API.
A GraphQL API is defined by a schema that describes everything that’s possible. The schema includes the types of queries, mutations and subscriptions the API supports.
For example, the schema for a vacation destination GraphQL API might support a query for a specific place. And that query takes an ID as an argument and returns a Place
object type:
type Query {
place(id: ID!): Place
}
The Place
object type might look something like this:
type Place {
id: ID!
name: String!
description: String
location: String!
maxGuests: Int!
perNight: Decimal!
wifi: Boolean!
bookings: [Booking]
reviews: [Review]
}
It has several scalar fields, and also indicates a relationship between place, bookings, and reviews.
So when you send in a query for a place, you can ask for any of these fields, like so:
query {
place(id: 3) {
name
location
perNight
bookings {
startDate
endDate
}
reviews {
rating
comment
}
}
}
How do GraphQL clients know what’s possible? Well, they can introspect the schema. In this 6-minute video, you’ll see how!
So, how do you go about implementing a GraphQL API in Elixir? Absinthe makes building a GraphQL API a breeze for Elixir developers. And that’s the topic we explore in the next video.
Unpack a Full-Stack GraphQL App Layer-By-Layer
Learn what it takes to put together a full-stack GraphQL app using Absinthe, Phoenix, and React in our Unpacked: Full-Stack GraphQL course. No need to piece together solutions yourself. Use this application as a springboard for creating your own GraphQL apps!
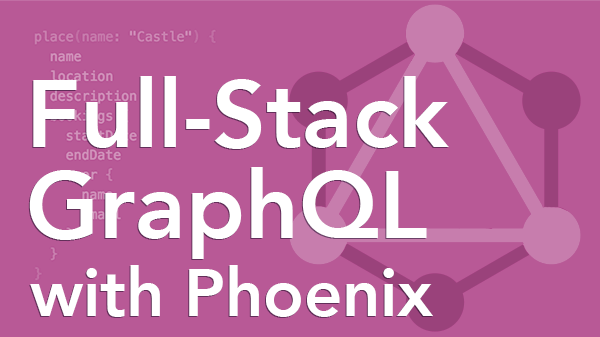