Transforming Data with Elixir
May 02, 2017
Elixir has really changed the way we think about programming. Instead of thinking about objects and calling methods that change their state, we now think more in terms of functions that transform data.
An HTTP web server is a great example of the Elixir way of doing things. At a high level, a web server takes a request and, through a series of transformations, produces a response. In this video from our new Developing With Elixir/OTP course, we set up a pipeline for those high-level transformations so we have a basic outline of our program.
The program doesn’t do anything interesting yet—the functions simply return hard-coded data. But we like to start this way as it helps us think through the program as a series of data transformations.
In the video, we started by sequencing the three transformation functions in a traditional style using intermediate variables:
def handle(request) do
conv = parse(request)
conv = route(conv)
format_response(conv)
end
You could condense this a bit and remove the temporary variables by nesting the function calls like so:
format_response(route(parse(request)))
But this is difficult to read since the functions resolve from the inside out. Using the pipe operator lets us unwind these nested calls by chaining the functions together in a more readable style:
request
|> parse
|> route
|> format_response
It transforms the request by piping the result of each function into the next function. Here’s a fun fact: At compile time this code is transformed to the nested call version.
If you’re making the change from OO programming to functional programming, one of the big differences is learning to think in terms of transforming data. You’ll see a lot more examples of this throughout the course.
In the next several modules, we’ll implement each of these functions so that an appropriate response is returned for different types of requests.
Build a complete Elixir/OTP app from start to finish!
Put Elixir and OTP into action as you build a concurrent, fault-tolerant application from scratch in our 6.5-hour Developing With Elixir/OTP course. By developing a real app with real code, you'll gain practical experience putting all the pieces together to craft applications the Elixir/OTP way.
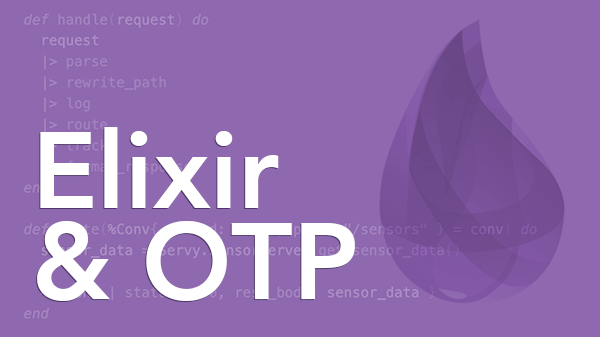