Map, Reduce, and Partition with Ruby
December 05, 2014
Ruby’s Enumerable
module is jam-packed with powerful methods. And knowing how to use them will instantly make you a more efficient Ruby programmer.
Four of the more common Enumerable methods for working with collections are select
, reject
, any?
, and detect
.
For example, let’s say we have an array of orders and we want to find all the big orders with totals greater than or equal to $300. We’d use select
like so:
orders.select { |o| o.total >= 300 }
Or we could toss out all the small orders with totals less than or equal to $300 using reject
:
orders.reject { |o| o.total <= 300 }
If we want to know if some of the orders have a status of pending, we could use any?
which returns true
or false
orders.any? { |o| o.status == :pending }
Or we could use detect
to get the first pending order:
orders.detect { |o| o.status == :pending }
But let’s take it up a notch!
Our Mastering Ruby Blocks course includes two videos on tapping into the power of the Enumerable module. Here’s the second video, in which we explore three methods that offer handy shortcuts for labor-intensive tasks: partition
, map
, and reduce
:
Want More?
Level up your Ruby skills and improve the design of your code by mastering Ruby blocks and iterators with 40+ code examples in our Mastering Ruby Blocks course.
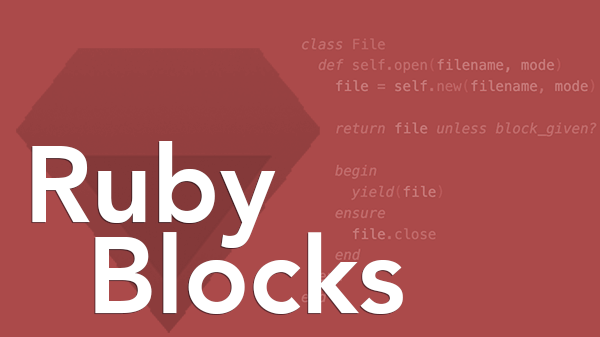